c++ - Your First Program On C++

Before we can write our first program (which we will do very soon), we need to know two things about development environments.
First, although our programs will be written inside .cpp files, the .cpp files themselves will be added to a project. Some IDEs call projects “workspaces”, or “solutions”. The project stores the names of all the code files we want to compile, and also saves various IDE settings. Every time we reopen the project, it will restore the state of the IDE to where we left off. When we choose to compile our program, the project tells the compiler and linker which files to compile and link. It is worth noting that project files for one IDE will not work in another IDE.
Second, there are different kinds of projects. When you create a new project, you will have to pick a project type. All of the projects that we will create in this tutorial will be console projects. A console project means that we are going to create programs that can be run from the dos or linux command-line. By default, console applications have no graphical user interface (GUI) and are compiled into stand-alone executable files. This is perfect for learning C++, because it keeps the complexity to a minimum.
Traditionally, the first program programmers write in a new language is the infamous hello world program, and we aren’t going to deprive you of that experience! You’ll thank us later. Maybe.
A quick note about examples containing code
Starting with this lesson, you will see many examples of C++ code presented. Most of these examples will look something like this:
1
2
3
4
5
6
7
8
| #include <iostream> int main() { using namespace std; cout << "Hello world!" << endl; return 0; } |
If you select the code from these examples with your mouse and then copy/past it into your compiler, you will also get the line numbers, which you will have to strip out manually. Instead, click the “copy to clipboard” link at the top of the example. This will copy the code to your clipboard without the line numbers, which you can then paste into your compiler without any editing required.
Visual Studio 2005 Express
To create a new project in Visual Studio 2005 Express, go to the File menu, and select New -> Project. A dialog box will pop up that looks like this:
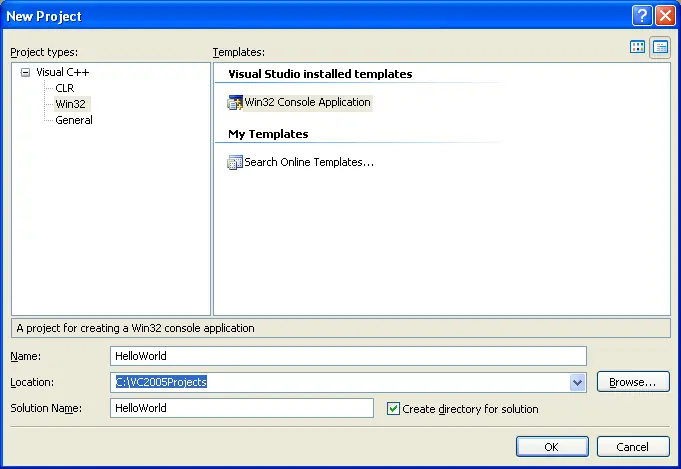
Select the Win32 project type, and Win32 Console Application will automatically be selected for you. In the Name field, you will enter the name of your program. Type in
HelloWorld
. In the Location field, pick a directory that you would like your project to be placed into. We recommend you place them in a subdirectory off of your C drive, such as C:\VC2005Projects
. Click OK, and then Finish.
On the left side, in the Solution Explorer, Visual Studio has created a number of files for you, including stdafx.h, HelloWorld.cpp, and stdafx.cpp.

In the text editor, you will see that VC2005 has already created some code for you. Select and delete the _tmain function, and then type/copy the following into your compiler:
1
2
3
4
5
6
7
8
| #include "stdafx.h" #include <iostream> int main() { std::cout << "Hello world!" << std::endl; return 0; } |
What you end up with should look like this:
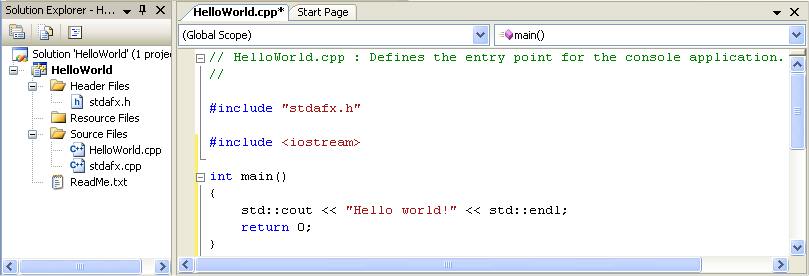
To compile your program, either press F7 or go to the Build menu and choose “Build Solution”. If all goes well, you should see the following appear in the Output window:
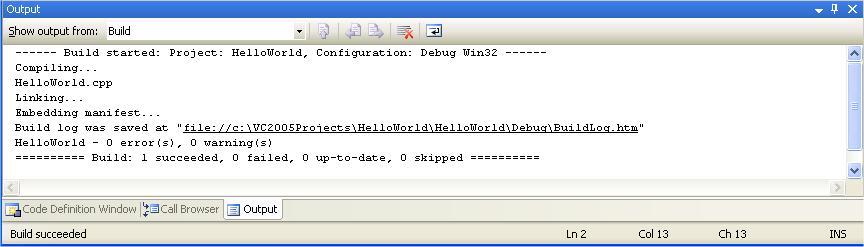
This means your compile was successful!
To run your compiled program, press ctrl-F5, or go the Debug menu and choose “Start Without Debugging”. You will see the following:
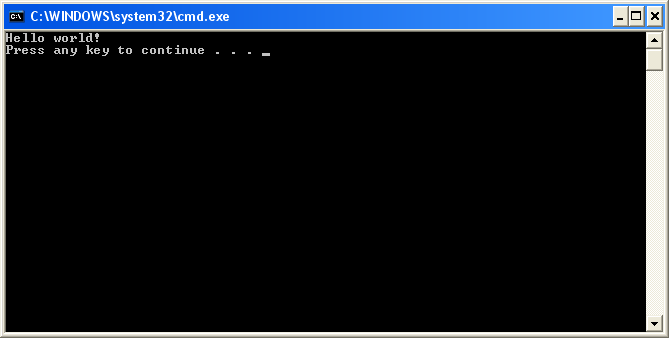
That is the result of your program!
Important note to Visual Studio users: Visual studio programs should ALWAYS begin with the following line:
Otherwise you will receive a compiler warning, such as
c:\test\test.cpp(21) : fatal error C1010: unexpected end of file while looking for precompiled header directive
Alternately, you can turn off precompiled headers. However, using precompiled headers will make your program compile much faster, so we recommend leaving them on unless you are developing a cross-platform program.
The example programs we show you throughout the tutorial will not include this line, because it is specific to your compiler.
Code::Blocks
To create a new project in Code::Blocks, go to the File menu, and select New Project. A dialog box will pop up that looks like this:

Select Console Application and press the Create button.
You will be asked to save your project. You can save it wherever you wish, though we recommend you save it in a subdirectory off of the C drive, such as
C:\CBProjects
. Name the project HelloWorld
.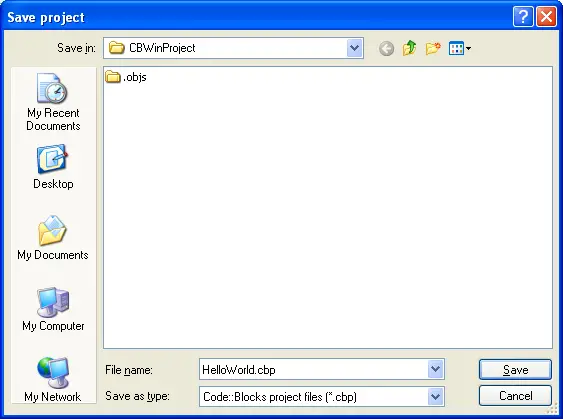
You will see “Console Application” under the default workspace:

Open the tree under “Console Application”, open “Sources”, and double click on “main.cpp”. You will see that the hello world program has already been written for you!
To build your project, press ctrl-F9, or go to the Build menu and choose “Build”. If all goes well, you should see the following appear in the Build log window:

This means your compile was successful!
To run your compiled program, press ctrl-F10, or go the Build menu and choose “Run”. You will see something similar to the following:
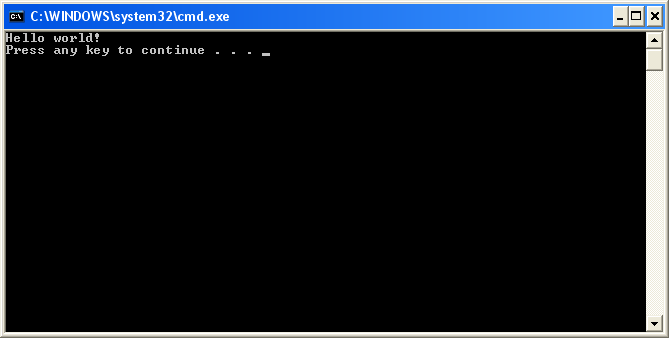
That is the result of your program!
Using a command-line based compiler
Paste the following into a text file named HelloWorld.cpp:
1
2
3
4
5
6
7
8
| #include <iostream> int main() { using namespace std; cout << "Hello world!" << endl; return 0; } |
From the command line, type:
g++ -o HelloWorld HelloWorld.cpp
This will compile and link HelloWorld.cpp. To run it, type:
HelloWorld
(or possibly .\HelloWorld
), and you will see the output of your program.
Other IDEs
You will have to figure out how to do the following on your own:
1) Create a console project
2) Add a .cpp file to the project (if necessary)
3) Paste the following code into the file:
1) Create a console project
2) Add a .cpp file to the project (if necessary)
3) Paste the following code into the file:
1
2
3
4
5
6
7
8
| #include <iostream> int main() { using namespace std; cout << "Hello world!" << endl; return 0; } |
4) Compile the project
5) Run the project
5) Run the project
If compiling fails
If compiling the above program fails, check to ensure that you’ve typed or pasted the code in correctly. The compiler’s error message may give you a clue as to where or what the problem is.
If you are using a much older C++ compiler, the compiler may give an error about not understanding how to include iostream. If this is the case, try the following program instead:
1
2
3
4
5
6
7
| #include <iostream.h> int main() { cout << "Hello world!" << endl; return 0; } |
In this case, you should upgrade your compiler to something more compliant with recent standards.
If your program runs but the window closes immediately
This is an issue with some compilers, such as Bloodshed’s Dev-C++. We present a solution to this problem in lesson 0.7 — a few common cpp problems.
Conclusion
Congratulations, you made it through the hardest part of this tutorial (installing the IDE and compiling your first program)! You are now ready to learn C++!
My Regards
Moataz Muhammed
Moataz Muhammed
No comments